브래의 슬기로운 코딩 생활
클래스 - 2, failable initializer, 클래스(class) 상속 본문
stored property와 computed property
computed property(계산 프로퍼티)는 property가 설정되거나 검색되는 시점에서 계산 또는 파생된 값
계산 프로퍼티 내에는
- 값을 리턴하는 게터(getter) 메서드
- 값을 대입하는 세터(setter) 메서드
class Man{
var age : Int = 1 // stored property
var weight : Double = 3.5 // stored property
var manAge : Int{ //메서드 같지만 computed property임
get{
return age-1
}
}
func display(){
print("나이=\(age), 몸무게=\(weight)")
}
init(age: Int, weight : Double){
self.age = age
self.weight = weight
}
}
var kim : Man = Man(age:10, weight:20.5)
kim.display()
print(kim.manAge)
// manAge는 계산 프로퍼티로 저장 프로퍼티 age의 값에서 1을 뺀 값으로 하겠다는 것임
computed property의 getter
manAge는 계산 프로퍼티로 저장 프로퍼티 age의 값에서 1을 뺀 값으로 하겠다.
setter가 없으면 get{ }는 생략할 수 있으며 변경하지 않더라도 var로 선언해야 함
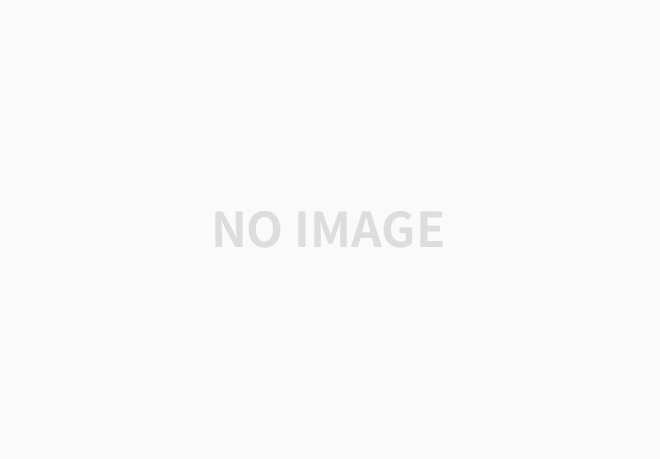
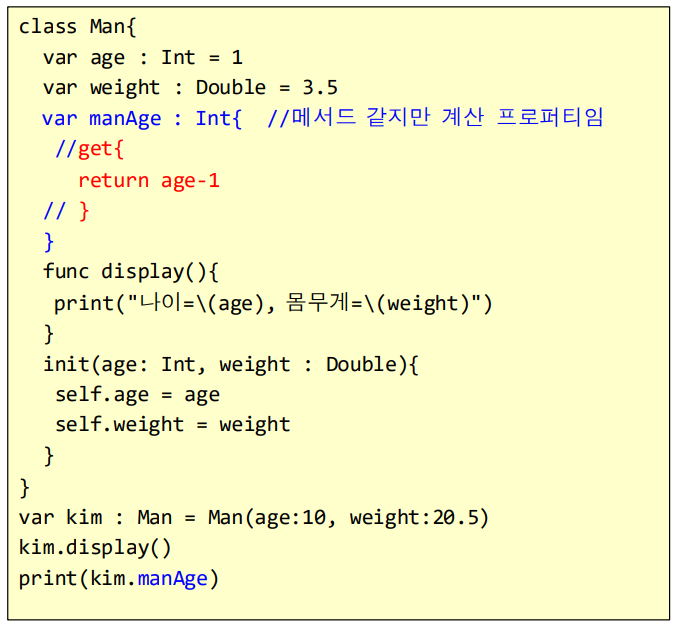
computed property의 setter
setter가 있으면 get{ }는 생략할 수 없음
매개변수명은 newValue가 기본
set(newValue){
age = newValue + 1
}
Shorthand Setter Declaration
- setter의 매개변수명이 newValue인 경우에만이렇게 “(newValue)” 생략 가능
set{
age = newValue + 1
}
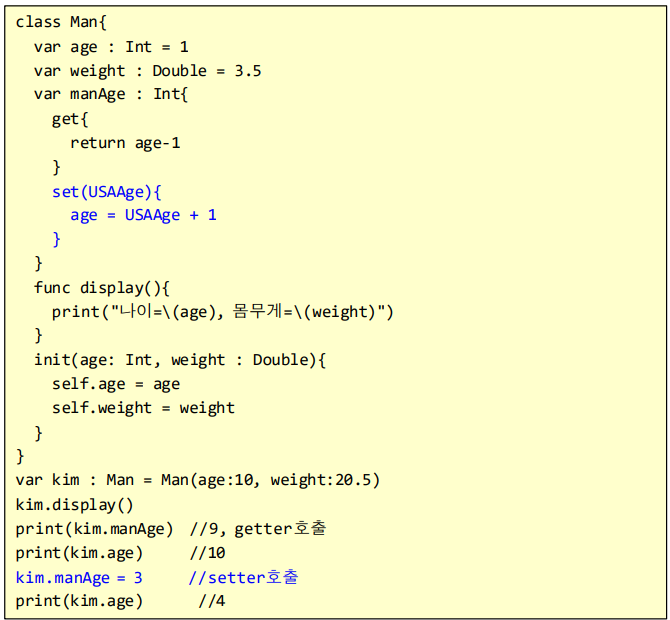
computed property의 getter와 setter
getter와 setter를 갖는 computed property manAge

method overloading : 생성자 중첩
매개변수의 개수와 자료형이 다른 같은 이름의 함수를 여러 개 정의
매개변수가 다른 두 생성자를 통해 두가지 방법으로 인스턴스를 만들 수 있음
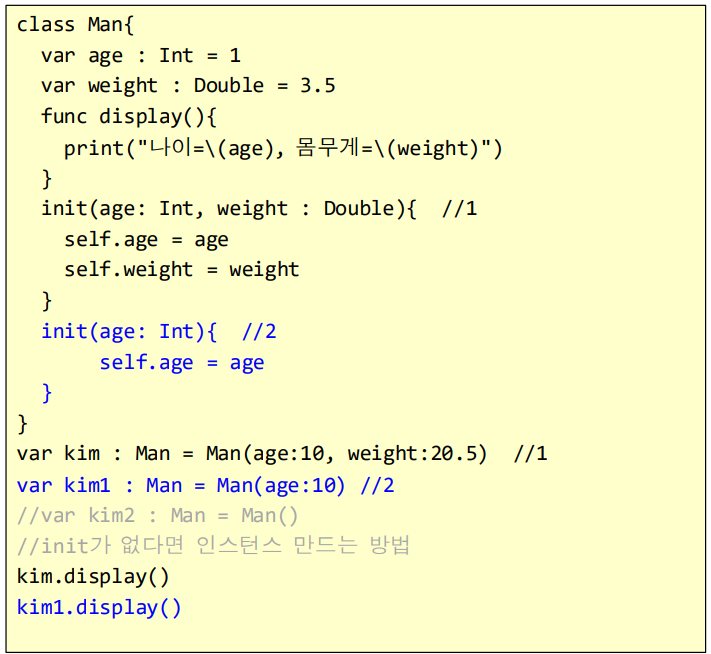
UIImage클래스의 init()함수 overloading
앱에서 이미지 데이터를 관리하는 클래스인 UIImage는 15개의 init()가 overloading되어 있음
let myImage: UIImage = UIImage(named: "apple.png")!
var aNewUIImage = UIImage(CGImage: imageRef)
1. Loading and Caching Images
init?(named: String, in: Bundle?, compatibleWith: UITraitCollection?)
init?(named: String)
init(imageLiteralResourceName: String)
2. Creating and Initializing Image Objects
init?(contentsOfFile: String)
init?(data: Data)
init?(data: Data, scale: CGFloat)
init(cgImage: CGImage)
init(cgImage: CGImage, scale: CGFloat, orientation: UIImage.Orientation)
init(ciImage: CIImage, scale: CGFloat, orientation: UIImage.Orientation)
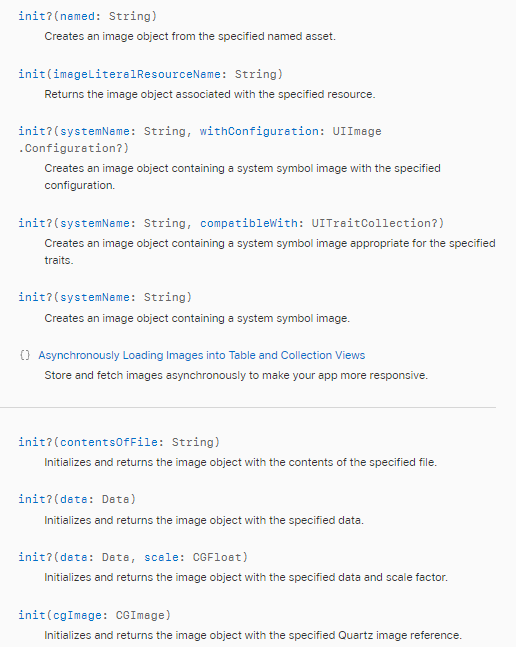
https://developer.apple.com/documentation/uikit/uiimage
Apple Developer Documentation
developer.apple.com
Failable Initializers(실패 가능한 생성자: init?)
let myImage: UIImage = UIImage(named: "apple.png")! //느낌표가 왜 있지?
- apple.png파일이 없으면 인스턴스를 만들 수 없고 nil
- nil값도 저장할 수 있으려면 init다음에 “?”를 하며 옵셔널 값이 리턴됨
init?(named name: String) // Failable Initializers
- init?로 만든 인스턴스는 옵셔널형으로 만들어져서, 사용하려면 옵셔널을 언래핑해야 해서 위의 예제에서 제일 마지막에 “!”가 있음

failable initializer(실패 가능한 생성자: init? init!)
init다음에 “?”나 “!” 하며 옵셔널 값이 리턴됨
오류 상황에 nil을 리턴하는 조건문이 있음
- return nil
init?
init!
failable initializer가 있는 클래스의 인스턴스 생성
- 오류
- error: value of optional type 'Man?' must be unwrapped to a value of type'Man'
- var kim : Man = Man(age:10, weight:20.5)
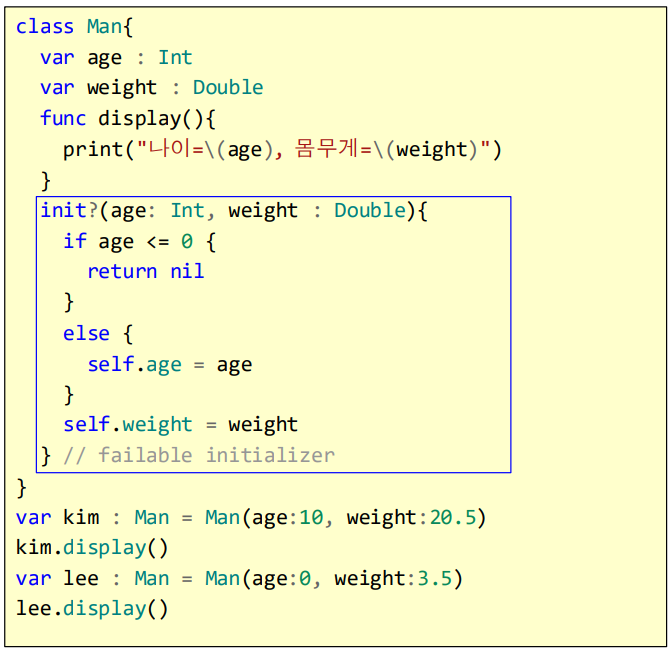

failable initialize가 nil반환
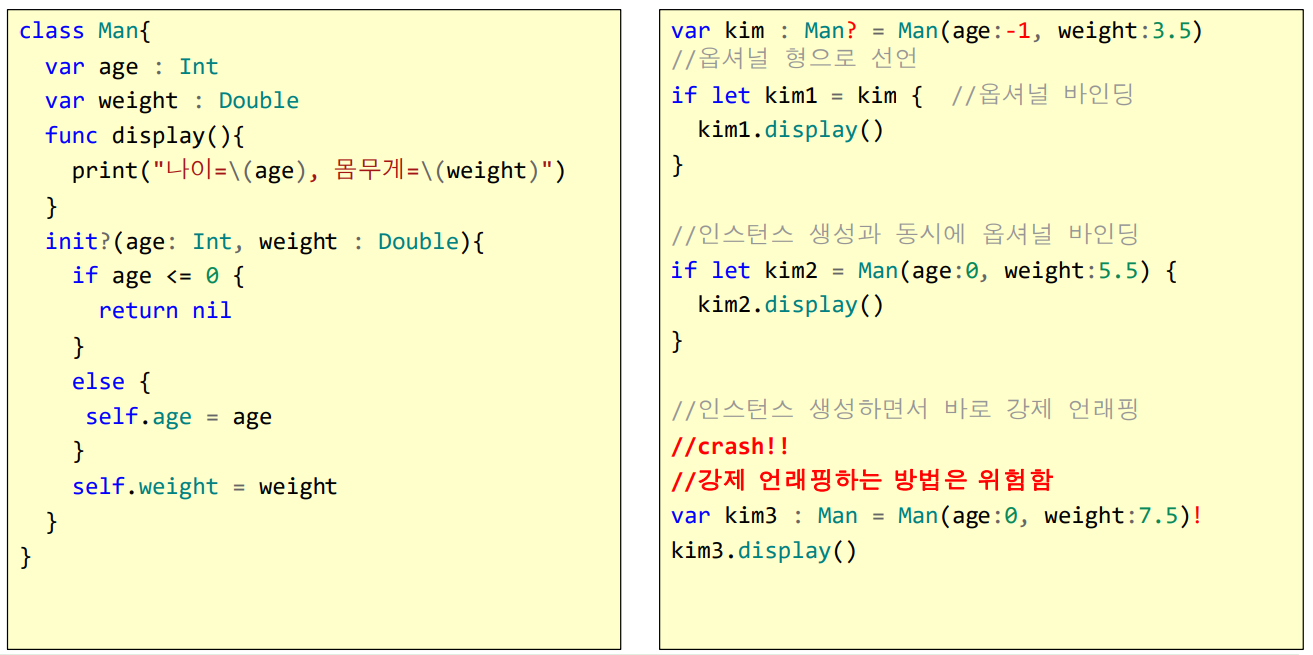
crash시 발생하는 오류와 .so파일
.so 나 .dylib
shared object
shared library
윈도우의 dll
동적 링크 라이브러리(프로그램 실행 시 필요할 때 연결)
.a
archive library
정적 링크 라이브러리
컴파일 시 포함됨

iOS UIKit component


클래스(class) 상속
superclass와 subclass

부모 클래스와 자식 클래스
상속된 클래스는 부모 클래스의 모든 기능을 상속받으며, 자신만의 기능을 추가
상속받은 클래스들을 하위 클래스(subclass) 또는 자식 클래스(child class)
하위 클래스가 상속받은 클래스는 부모 클래스(parent class) 또는 상위 클래스(super class)
단일 상속 (single inheritance)
- Swift에서 하위 클래스는 단 하나의 부모 클래스만 상속받을 수 있음

스위프트 상속
class 자식:부모 {
}
- 부모 클래스는 하나만 가능
- 콜론 다음이 여러 개이면 나머지는 프로토콜
class 클래스명:부모명, 프로토콜명{}
- 부모가 있으면 부모 다음에 표기
class 클래스명:부모명, 프로토콜명1,프로토콜명2 {}
class 클래스명:프로토콜명{}
- 부모가 없으면 바로 표기 가능
class 클래스명:프로토콜명1, 프로토콜명2{}
상속은 클래스만 가능
클래스, 구조체(struct), 열거형(enum), extension에 프로토콜을 채택(adopt)할 수 있다.

상속 : 부모가 가진 것을 물려받아요.

super : 부모 메서드 호출 시 사용
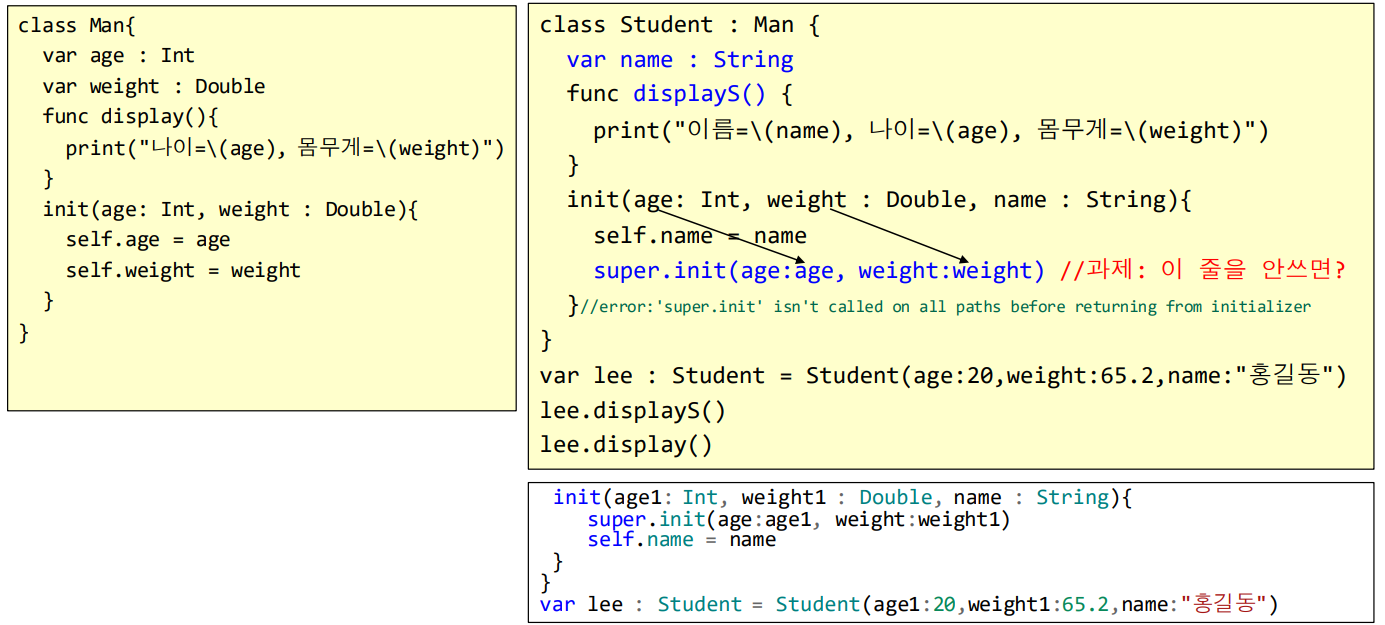
override : 부모와 자식에 같은 메서드가 있으면 자식 우선
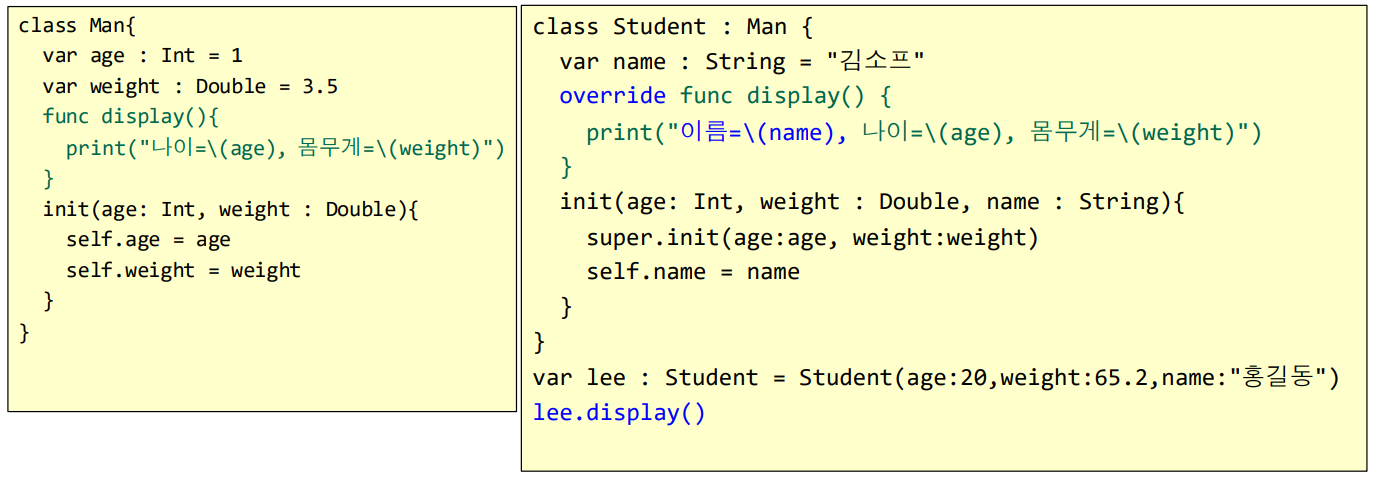
- 부모와 자식에 display()라는 메서드가 있어서 Student클래스는 display() 메서드가 두 개임
- Student클래스의 인스턴스 lee가 display()를 호출할 때, 자식클래스가 새로 만든 display() 메서드가 우선적으로 호출되려면 func 앞에 override키워드 씀
'Class > Swift' 카테고리의 다른 글
Xcode 사용법 - 1 (2) | 2023.01.24 |
---|---|
extension, Swift 접근 제어 (access control, access modifier), 프로토콜(protocol)과 Delegate, 열거형 (enum) (0) | 2023.01.23 |
클래스 (class) - 1 (0) | 2023.01.13 |
1급 객체(first class object), 1급 시민(first class citizen), 클로저(Closure) (0) | 2023.01.12 |
함수와 메서드(method) (2) | 2023.01.11 |